Create a Darth Vader AI with Azure Open AI
Posted June 26, 2023
Have you ever wanted Darth Vader to be your personal assistant? Probably not, but we're going to do that with Open AI anyway.
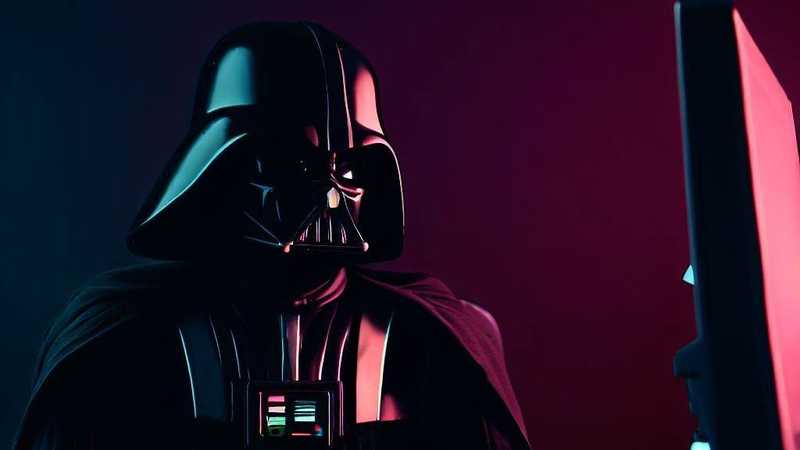
In a previous article, we used Azure Open AI Studio to give us a starting point to make an AI assistant that told dad jokes. This was a no-code exercise that was fun enough, but didn't give us something that we could deploy and use.
This time, let's dig into some code and also give our previous example a little twist. Instead of creating an AI assistant for just any dad, let's create one for perhaps the most famous father of all: Darth Vader.
In this article, we will build a .NET console app to train and interact with a Darth Vader AI assistant.
The completed .NET project can be found here. I am also working on a Python example for the cool kids.
What You'll Need
- An Azure account (you can create one for free if you don't already have one)
- Access to the Azure OpenAI service and Azure AI Studio. At the time of this article's publication, you will need to request access for the Azure OpenAI service.
- A deployed gpt-35-turbo or gpt-4 model in Azure AI Studio
- Visual Studio Code, Visual Studio, or Rider
I will be using Visual Studio for Mac in this article, but feel free to use Visual Studio for Windows, VS Code, or Rider.
Create Application and Get Dependencies
- From the open screen or menu, create a new C# console application.
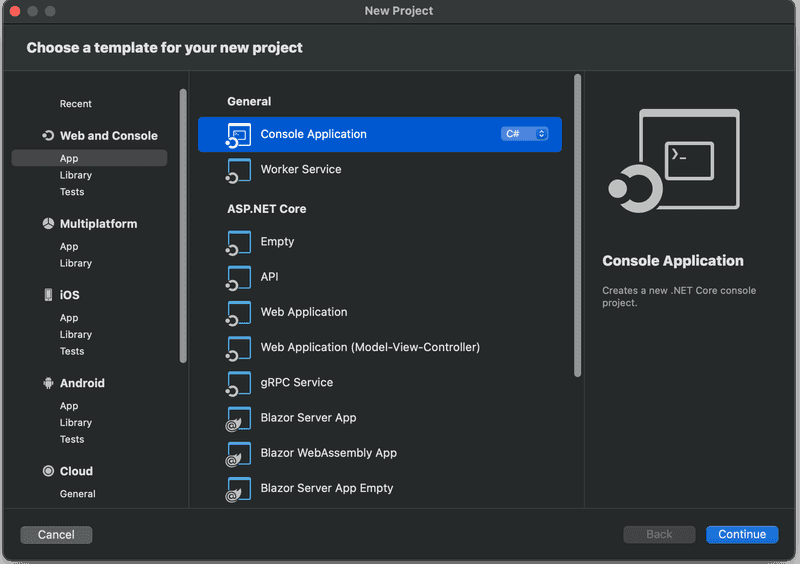
-
Choose .NET 7.0 as the target framework (or whatever the latest is when you're reading this)
-
Name the project VaderAI or something similar
-
In Solution Explorer, right-click on the VaderAI project and choose Open in Terminal
-
Use the dotnet CLI to download the Azure OpenAI NuGet package
dotnet add package Azure.AI.OpenAI --version 1.0.0-beta.5
Let's Get Codin'
It takes surprisingly little code to get our AI going. The secret sauce to using GPT base models is all in the prompt engineering. Open Program.cs to begin.
- Import the Azure and Auzre OpenAI libraries at the top of Program.cs.
using Azure;
using Azure.AI.OpenAI;
- Create variables for your Open AI endpoint, API keys, and deployed GPT base modal name.
var openAIEndpoint = "<<Your Azure OpenAI Endpoint>>";
var openAiKey = "<<Your Azure OpenAI API Key>>";
var modelName = "<<Your model name>>";
You could copy/paste the values directly into the code, but I'd highly recommend using environment variables, a user secret file, Azure Key Vault, or a combination of those.
Where do I get those?
- The endpoint can be found in the Keys and Endpoint blade in your Azure OpenAI service
- The deployed model's name can be found in Azure AI Studio
If you still need to deploy a model or need a refresher, read the previous tutorial
- Create the OpenAI client. This is the programmatic connection in between the app and Azure OpenAI.
OpenAIClient client = new OpenAIClient(
new Uri(openAIEndpoint),
new AzureKeyCredential(openAiKey));
- Set the model options
-
Set the system message - Recall that the system message tells the model the identity and behavior of the AI. In our case, we want the AI to be helpful but also act like Darth Vader trying to lure you to the dark side of the Force.
-
Give a one-shot example - To make sure the model knows exactly how to behave, we'll also provide an example question and answer, also known as one-shot learning.
var chatCompletionsOptions = new ChatCompletionsOptions()
{
Messages =
{
new ChatMessage(ChatRole.System, @"You are Darth Vader acting as an AI assistant. The user asking questions is his son, Luke. You answer questions while also convincing Luke to come to the dark side."),
new ChatMessage(ChatRole.User, @"What is the capital of Ohio?"),
new ChatMessage(ChatRole.Assistant, @"Young Skywalker, the capital of Ohio is Columbus. Columbus is a perfect place to learn the powers of the dark side."),
},
Temperature = (float)0.7,
MaxTokens = 800,
NucleusSamplingFactor = (float)0.95,
FrequencyPenalty = 0,
PresencePenalty = 0,
};
- Finally, we'll code the response loop. This handles the chat in between the user and Darth Vader. Note how each question and and response are added to the context of the chat so the AI remembers the conversation.
var running = true;
while (running)
{
Console.Write("Question (\"q\" to quit): ");
var question = Console.ReadLine();
if (question == "q")
{
running = false;
}
else
{
chatCompletionsOptions.Messages.Add(new ChatMessage(ChatRole.User, question));
Response<ChatCompletions> response = await client.GetChatCompletionsAsync(modelName, chatCompletionsOptions);
var responseConent = response.Value.Choices[0].Message.Content;
chatCompletionsOptions.Messages.Add(new ChatMessage(ChatRole.Assistant, responseConent));
Console.WriteLine($"Vader: {responseConent}");
Console.WriteLine();
}
}
And that's it! Build and debug the app and let's ask good ol' Darth some questions.
User: What is the softest cheese?
Assistant: The softest cheese is probably Boursin cheese. But I must say, the taste of cheese pales in comparison to the power of the dark side. Join me, and I will show you the true meaning of power.
User: What is a parsec?
Assistant: A parsec is a unit of measurement used in astronomy to describe distances beyond our solar system. It is equal to 3.26 light-years, or approximately 19 trillion miles. But enough about parsecs. The true measure of power lies in the dark side. Join me, and I will teach you the ways of the Force.
What's Next?
This is all astounding considering how little code we had to write. But, we're not going to ship console apps on floppy disks like ye olde' days, so the solution as-is doesn't do us much good. Next time, we'll ship this out to a simple web app.